Accounts
Your Account Key can be found within the Admin portal under the Developers
>> Embed
menu.
Creating Accounts
You can create a new account via a POST request (using basic auth) to https://admin.myintranetapps.com/app/api.php with an "Account-Key" header set to "XXXX" (where XXXX is your account key) that contains a JSON body that looks like:
{
"name": "new client name",
"package_id": "9",
"active": "1",
"email_admin": "newaccountadmin@email.com",
"password": "newadminpassword",
"subdomain": "example",
"type": "standard",
"domain": "www.redirect.com",
"datashare": "1",
"first_name": "Jane",
"last_name": "Doe",
"environment": "sandbox"
}
Parameter | Definition |
---|---|
| The human readable name of the company you are adding an account for, typically the legal name. This is displayed to the user. |
| Specifies an identifier for a collection of account settings called a `package`. If no |
| A flag that indicates you want this account to be active on creation. Either 0 or 1. |
| Address that indicates the email we should use for communication to the manager of the account, reasons for sending an email might include sending system service/maintenance notices or requests to re-authorize an integration if access has been revoked for some reason. |
| Used to allow the default "admin" account to login with the given password, leave this blank to disable admin login. |
| Required as each new account is accessible under its own subdomain of myintranetapps.com, the text you specify here will be used to create a domain like "my-company.myintranetapps.com". Must be 50 characters or less and use alphanumeric characters and dashes only. If the subdomain is already in use by another account, the request will fail with a 409 response:
CODE
In this case, the request should be retried with a new subdomain. |
| Indicates the account type which is either "reseller" or "standard", for your purposes you should leave this as “standard”. |
| An optional field used when you want to add a custom (DNS CNAME) domain name to the system instead of using the myintranetapps.com domain, you shouldn't need this field and can leave blank. If you have not previously setup a DNS CNAME entry for your custom domain do not provide a value in this field or the account creation will not succeed. |
| Indicates that you want the account setup with permissions already active to allow full sharing with the master account. Default is to have sharing on. If you do not want the account to share data with you, be sure to set the value to 0 for your use case. |
| Sets the first name of the “admin” user assigned to the account. Defaults to “Admin” if not specified in the request’s body and can be changed later in the Admin portal. |
| Sets the last name of the “admin” user assigned to the account. Defaults to “Admin” if not specified in the request’s body and can be changed later in the Admin portal. |
| Possible values: [“dev”, “integration”, “sandbox”, “production”]. If not set, it defaults to the same environment as the parent account. |
The response you get back will be in JSON and look like:
{
"status_code":200,
"message":"Account successfully created",
"account_key":"<<<UNIQUE_KEY>>>",
"subdomain":"example",
"account_domain":"example.myintranetapps.com",
"name":"new client name",
"admin_email":"newaccountadmin@email.com"
}
You should save the unique key for future reference in your system, you will use this unique account key whenever you need to interact with this account in the future
If something goes wrong while processing your POST request ("status_code" value will differ from 200), the "message" property will contain an error description.
Error responses
HTTP response status | Error message | Description |
---|---|---|
400 | Bad Request | Check that you are sending a standard “Host” header in your request |
401 | Missing username/API Key from Basic Auth header | |
401 | Missing 'Account-Key' request header | |
403 | Invalid or expired API Key | If API Key used |
403 | Invalid API Key | If API Key used |
401 | Missing password from Basic Auth header | If username/password used |
403 | Invalid username/account key | If username/password used |
403 | Invalid password | If username/password used |
403 | Operation is not permitted - missing scope: "admin.account.create" | Please check your API Key contains required scope. |
403 | Parent account key must be used when making a 'create account' request. Child account key given. Please check that correct key is used in Account-Key header | |
400 | Missing or empty required fields: {fields list} | Missing one or more required fields: 'type', 'name', 'active', 'subdomain', 'email_admin', 'password' |
403 | Invalid subdomain, must be 50 characters or less and use alphanumeric characters and dashes only | |
409 | Subdomain already in use | |
409 | Custom domain already in use | |
409 | Invalid package_id | |
409 | Invalid environment | |
409 | Account creation failure - accounts | This is internal error during account creation. |
409 | Account creation failure - users | This is internal error during user creation |
409 | Account creation failure - default package | This is internal error during package creation |
Listing Accounts
You can list all accounts previously created via a POST request (using basic auth) to https://admin.myintranetapps.com/app/api.php with an "Account-Key" header set to "XXXX" (where XXXX is your account key) that contains a JSON body that looks like:
{
"action": "list_accounts"
}
The response you get back will be in JSON and look like:
{
"status_code":200,
"message": "Accounts list successfully generated",
"accounts":[
{
"name": "General Company Ltd",
"key": "5a70d8fcd44aa9.76285892",
"subdomain": "generalco",
"domain": "",
"package_id": "93",
"email_admin": "cfo@example.com"
}
]
}
If something goes wrong while processing your POST request ("status_code" value will differ from 200), the "message" property will contain an error description.
Error responses
HTTP response status | Error message | Description |
---|---|---|
400 | Bad Request | Check that you are sending a standard “Host” header in your request |
401 | Missing username/API Key from Basic Auth header | |
401 | Missing 'Account-Key' request header | |
403 | Invalid or expired API Key | If API Key used |
403 | Invalid API Key | If API Key used |
401 | Missing password from Basic Auth header | If username/password used |
403 | Invalid username/account key | If username/password used |
403 | Invalid password | If username/password used |
403 | Operation is not permitted - missing scope: "admin.account.list" | Please check your API Key contains required scope. |
409 | Account list failure | This is internal error when tried to get list of account |
Updating Accounts
You can update an account previously created via a POST request (using basic auth) to https://admin.myintranetapps.com/app/api.php with an "Account-Key" header set to "XXXX" (where XXXX is your account key) that contains a JSON body that looks like:
{
"action": "update_account",
"account_key": "5a70d8fcd44aa9.76285892",
"package_id": "9",
"active": "1",
"email_admin": "newaccountadmin@email.com"
}
Property called "account_key" must be set to the value of the account key of the account to update. Following properties of the JSON posted are optional: "package_id", "active", "email_admin", but there must be at least one property set to perform an update.
The response you get back will be in JSON and look like:
{
"status_code":200,
"message":"Account successfully updated",
"account_key": "5a70d8fcd44aa9.76285892"
}
If something goes wrong while processing your POST request ("status_code" value will differ from 200), the "message" property will contain an error description.
Error responses
HTTP response status | Error message | Description |
---|---|---|
400 | Bad Request | Check that you are sending a standard “Host” header in your request |
401 | Missing username/API Key from Basic Auth header | |
401 | Missing 'Account-Key' request header | |
403 | Invalid or expired API Key | If API Key used |
403 | Invalid API Key | If API Key used |
401 | Missing password from Basic Auth header | If username/password used |
403 | Invalid username/account key | If username/password used |
403 | Invalid password | If username/password used |
403 | Operation is not permitted - missing scope: "admin.account.update" | Please check your API Key contains required scope. |
400 | No account_key is set for update | |
400 | No fields to update. Allowed fields: package_id, active, email_admin | |
409 | Account did not updated | This is internal error when during update account |
Multi-level Accounts
Multi level accounts can be used by service vendors to provide a branded experience to the financial services firms they work with and in turn their business customers.
To enable multi-level accounts you will need to make a request to our support help desk
Account levels:
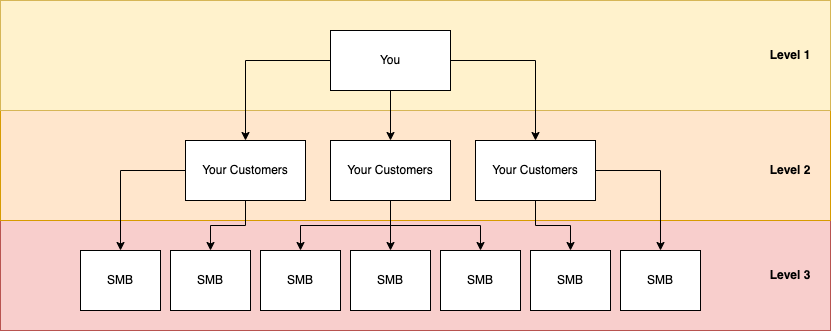
Some things are done differently with multi-level accounts and are noted here:
When creating an account the
type
parameter should be set to a value ofreseller
After creating an account for your customer you should create and store an API key for their account and use this to conduct actions on their account or their SMB customers.
Accounts are managed in a parent-child relationship, generally child accounts will inherit configuration from their parent account