Link Page Element Widget
The Link Page Element Widget is used if you want to present forms, dashboards, insights, ratios, workflows and other page elements to gather data or inform your end users OR you want to present these to your own team. Banking and Tax consent are also gathered via this mode.
To present forms, insights and ratios (collectively known as page elements) you need to:
Provision an account to store your customers data
Request an embed token from the API
Display a specific page element via the Link Page Element Widget
Either use the API to pull captured information OR display pre-built dashboard elements within your own software application UI.
Provision an Account
Before a customer can begin to share information a customer account needs to be provisioned to hold data synchronized for that specific business customer. To create a new account you need to make a POST request to the account administration API.
As part of the account creation you will specify a secret password/key which you will need to store and reference in subsequent steps. You will also need to specify a subdomain, which should be generated with a reasonable degree of uniqueness because the account creation request will fail if the provided subdomain is already in use. The response from successfully creating an account will contain account_domain
, subdomain
and account_key
fields that you should store for subsequent reference.
Link Page Element Widget
The Link Page Element widget can display portions of the Boss Insights platform within your own software, either to your end users or in a back office portal your team accesses, e.g. as a provider of financial capital to small business I may embed the consent form for gathering tax records from my end users, then using another embed show my credit approval team specific ratios or document downloads based on information gathered.
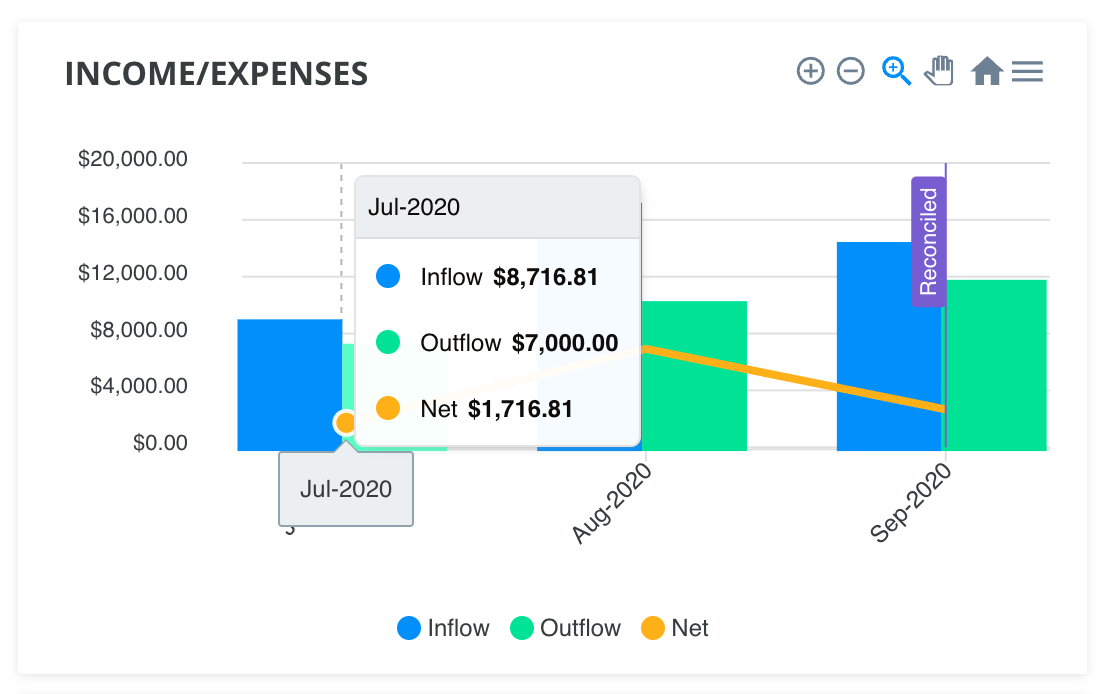
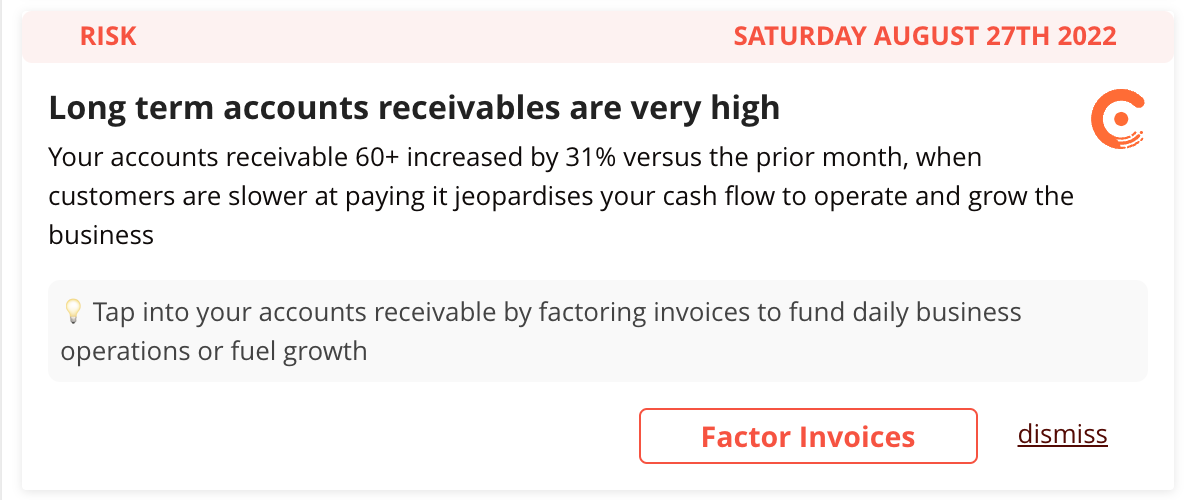
Before a page element can be embedded an embed token must be requested via API, this token provides authorization to the Link Page Element widget.
Embedding
To embed the Link Page Element widget in to your web application you need to include a reference to a JavaScript file and create an HTML DIV tag within your page to render the widget within. This DIV tag will contain the page element.
The container DIV tag:
<div id="link_page_element"></div>
We recommend styling this containing element with CSS to set the width and height
The widget embed script:
<script type="text/javascript" src="https://example.myintranetapps.com/dock/link/2022-09-08/link.js"></script>
<script>
var widget = new DockLink({
container: document.getElementById('link_page_element'),
domain: "ACCOUNT_DOMAIN",
token: "EMBED_TOKEN",
app: "company/forms",
options:{
key: "taxstatus"
},
onSuccess: function (data) {
console.log('calling onSuccess callback with this data: ', data);
},
onError: function (data) {
console.log('calling onError callback with this data: ', data);
},
onEvent: function (data) {
console.log('calling onEvent callback with this data: ', data);
}
});
widget.render();
</script>
The domain of the
src="https://example.myintranetapps.com/dock/link/2022-09-08/link.js"
attribute should be changed to your domain. (Replaceexample.myintranetapps.com
with your domain).When you request an embed token, the API response contains an
account_domain
value that should replaceACCOUNT_DOMAIN
, the response also includes a token value, use it to replaceEMBED_TOKEN
.
After embedding the widget you may receive warnings in your browsers developer console, see the Browser Security section to understand some issues that can impact embedded widgets
The app
parameter dictates the type of element that will be embedded, while options
relate to the app and will vary depending on the app chosen.
Events
When an end user interacts with a widget certain events will be raised to your code to handle or ignore. the onEvent callback function you define will be provided with the event type and other properties depending on the event. For example when embedding a form for an end user to fill out an event will be raised when they have successfully submitted the form - you can then take appropriate actions via your javascript function such as updating progress indicators or redirecting the users browser to the next step in your software.
Note the onSuccess and onError callback functions are optional, if omitted the onEvent callback will be used.
Example Configurations
The following examples show some typical use cases for the Link Page Element widget. Each example requires a different type of Embed Token.
Example 1: Access your own account for a back office dashboard
<script type="text/javascript" src="https://example.myintranetapps.com/dock/link/2022-09-08/link.js"></script>
<script>
var widget = new DockLink({
container: document.getElementById('link_page_element'),
domain: "ACCOUNT_DOMAIN", // your domain
token: "EMBED_TOKEN", // token to access your own account
app: "dashboard/insights-dashboard",
options: {
accountKey: '632364a5a046f7.42267761', // key of customer account (required)
}
});
widget.render();
</script>
Additional available options for “dashboard/insights-dashboard” app:
key | example value | description |
---|---|---|
tabs | “insights,accounting” | A comma-separated whitelist of dashboard tab ids. Typical IDs include: |
hide | “welcome,metrics” | A comma-separated list of dashboard elements you wish to hide |
Example 2: Access a customer account so an end-user can fill in a form
<script type="text/javascript" src="https://example.myintranetapps.com/dock/link/2022-09-08/link.js"></script>
<script>
var widget = new DockLink({
container: document.getElementById('link_page_element'),
domain: "ACCOUNT_DOMAIN", // customer domain
token: "EMBED_TOKEN", // token to access a customer account
app: "company/forms",
options: {
key: 'taxstatus' // the form to display
},
});
widget.render();
</script>
Example 3: Access your own account and review a customer’s form
<script type="text/javascript" src="https://example.myintranetapps.com/dock/link/2022-09-08/link.js"></script>
<script>
var widget = new DockLink({
container: document.getElementById('link_page_element'),
domain: "ACCOUNT_DOMAIN", // your domain
token: "EMBED_TOKEN", // token to access your own account and view a customer’s pages
app: "company/forms",
options: {
key: 'taxstatus' // the form to display
},
});
widget.render();
</script>
Example 4: Gather bank account consent
<script type="text/javascript" src="https://example.myintranetapps.com/dock/link/2022-09-08/link.js"></script>
<script>
var widget = new DockLink({
container: document.getElementById('link_page_element'),
domain: "{{ ACCOUNT_URL }}",
token: "{{ EMBED_TOKEN }}", // token to access your own account
app: "bank-mx",
cancel: "{{ SITE_URL }}/cancel.html",
redirect: "{{ SITE_URL }}/finished.html",
});
widget.render();
</script>
Bank options for the app
value are bank-mx
or bank-yodlee
Browser Security
Do not embed the widget within a html iframe, many integrations utilize OAuth and based on OAuth specs will block loading when requested from within a frame.
CORS Access
If you get errors in your browser about Access-Control-Allow-Origin you need to add the domain name you will be embedding the widget from to the allowed list of URLs. In the admin portal go to the “Embed” menu and then add the URL for your app and click save, the URL will then be added to a list that is allowed to embed the widget
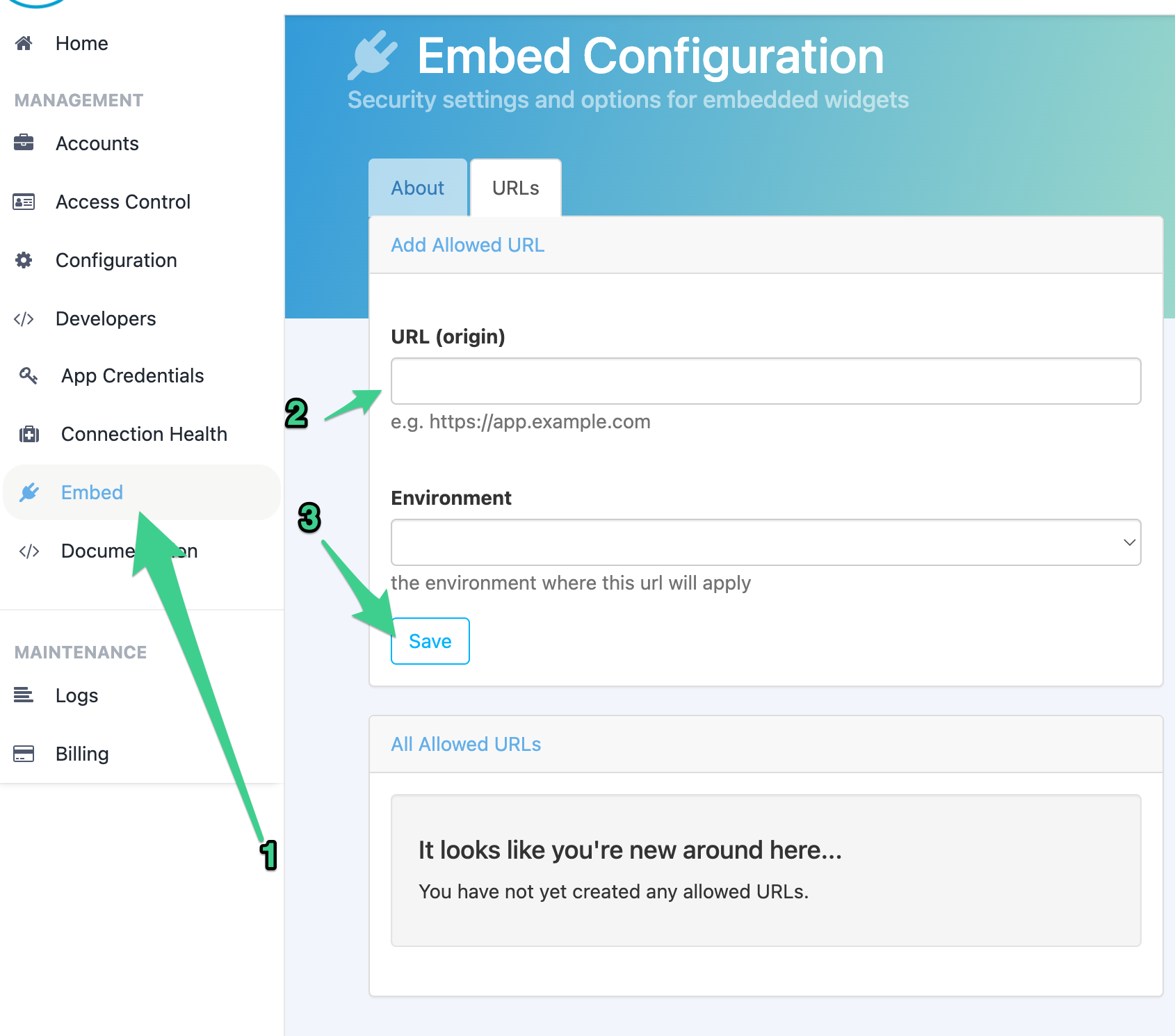
Content Security Policy (CSP)
Embedding the link widget will result in external resources being loaded in to your page. These resources will come from your Boss Insights domain e.g. example.myintranet.apps , if you use a content security policy (CSP) header within your application you will want to add this domain to the list of allowed domains that can reference resources. Depending on widget configuration additional scripts, frames, images and stylesheets may be loaded.
Framing
The widget should not be embedded within a frame, many integrations utilize OAuth, the spec for which recommends blocking attempts to load inside of a frame.

If you see browser console errors related to framing ensure you are not loading the widget within a frameset.